PDF Tool APIサンプル集:矩形内で折り返す文字列
PDF出力する元ファイル(入力ファイル)パス、PDFの出力先となるファイルパスを指定して
矩形内で折り返す文字列を書きこんだPDFを出力するコンソールアプリケーションです。
概要
コマンドラインでの実行例
sample.exe c:\test\test.pdf c:\sav\out.pdf
ダウンロード
出力結果イメージ
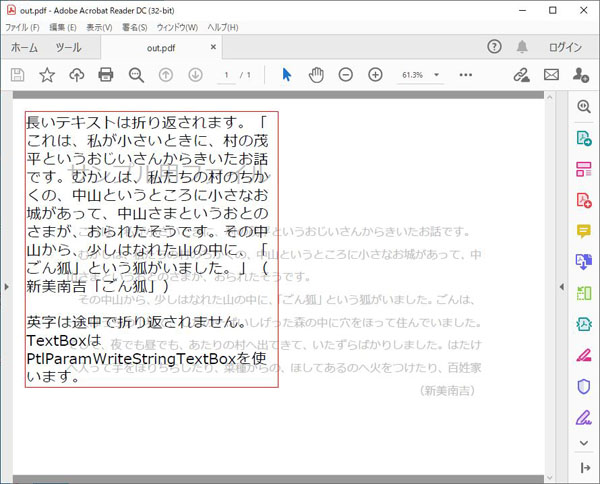
サンプルコード
/* Antenna House PDF Tool API 7.0 C# Interface sample program 概要:矩形内で折り返す文字列 Copyright 2021 Antenna House,Inc. */ using System; using PdfTkNet; namespace sample03cs { class Program { static void Main(string[] args) { Console.WriteLine("PDF Tool API V7.0 C# サンプル"); // 入出力ファイル名と設定ファイル名の初期値を設定 string inFilePath = @"C:\test\test.pdf"; string outFilePath = @"C:\sav\out.pdf"; // 入力ファイル名 if (args.Length > 0) { inFilePath = args[0]; } // 出力ファイル名 if (args.Length > 1) { outFilePath = args[1]; } try { using (PtlParamInput input = new PtlParamInput(inFilePath)) using (PtlParamOutput output = new PtlParamOutput(outFilePath)) using (PtlPDFDocument doc = new PtlPDFDocument()) using (var option = new PtlOption()) { option.setUnit(PtlOption.UNIT.UNIT_PT); doc.load(input); string fontName = "メイリオ"; float fontSize = 24.0f; using (PtlParamFont fontNormal = new PtlParamFont(fontName, fontSize, false, false, true)) using (PtlParamFont fontBoldItalic = new PtlParamFont(fontName, fontSize, true, true, true)) using (PtlColorDeviceRGB colorBlack = new PtlColorDeviceRGB(0.0f, 0.0f, 0.0f)) using (PtlColorDeviceRGB colorRed = new PtlColorDeviceRGB(1.0f, 0.0f, 0.0f)) { using (var pages = doc.getPages()) using (var page = pages.get(0)) //1ページ目 using (var pageSize = page.getSize()) using (var content = page.getContent()) using (PtlRect rect = new PtlRect(20, 20, pageSize.getWidth() - 20, pageSize.getHeight() - 20)) using (PtlTextBox textBox = content.drawTextBox(rect, PtlContent.ALIGN.ALIGN_TOP_LEFT, 400, 400)) { // TextBoxの縁取りを付ける textBox.setOutlineColor(colorRed); // 縁取りがテキストを囲むサイズに変わるよう指定 textBox.fitToBBox(true); using (PtlParamWriteStringTextBox paramWriteString = new PtlParamWriteStringTextBox()) { paramWriteString.setFont(fontNormal); paramWriteString.setTextColor(colorBlack); string text1 = "長いテキストは折り返されます。"; textBox.writeString(text1, paramWriteString); // 文字列を出力 string text2 = "「これは、私が小さいときに、村の茂平というおじいさんからきいたお話です。むかしは、私たちの村のちかくの、中山というところに小さなお城があって、中山さまというおとのさまが、おられたそうです。その中山から、少しはなれた山の中に、「ごん狐」という狐がいました。」(新美南吉「ごん狐」)"; textBox.writeStringNL(text2, paramWriteString); // 文字列を出力して改行 textBox.writeNL(); // 改行 string text3 = ("英字は途中で折り返されません。TextBoxはPtlParamWriteStringTextBoxを使います。"); textBox.writeStringNL(text3, paramWriteString); // 文字列を出力して改行 } textBox.terminate(); doc.save(output); Console.WriteLine("-- 完了 --"); } } } } catch (PtlException pex) { Console.WriteLine(pex.getErrorCode() + " : " + pex.getErrorMessageJP()); pex.Dispose(); } catch (Exception ex) { Console.WriteLine(ex.Message); } } } }