PDF Tool APIサンプル集:下線付き文字列・取り消し線付き文字列・太字、斜体の文字列・枠で囲った文字列(背景色あり)
PDFの出力先となるファイルパスを指定して
下線付き文字列・取り消し線付き文字列・太字、斜体の文字列・枠で囲った文字列(背景色あり)を書きこんだPDFを出力するコンソールアプリケーションです。
概要
コマンドラインでの実行例
sample.exe c:\sav\out.pdf
ダウンロード
出力結果イメージ
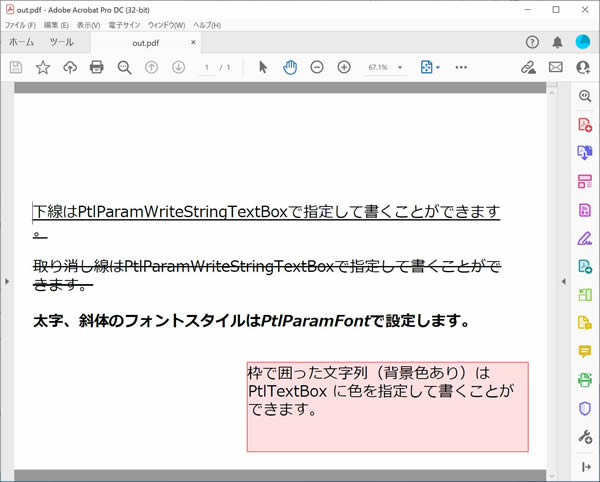
サンプルコード
/* Antenna House PDF Tool API 7.0 C# Interface sample program 概要:下線付き文字列・取り消し線付き文字列・太字、斜体の文字列・枠で囲った文字列(背景色あり) Copyright 2021 Antenna House,Inc. */ using System; using PdfTkNet; namespace sample02cs { class Program { static void Main(string[] args) { Console.WriteLine("PDF Tool API V7.0 C# サンプル"); // 出力ファイル名の初期値を設定 string outFilePath = @"C:\sav\out.pdf"; // 出力ファイル名 if (args.Length > 0) { outFilePath = args[0]; } try { using (PtlParamOutput output = new PtlParamOutput(outFilePath)) using (PtlPDFDocument doc = new PtlPDFDocument()) using (var option = new PtlOption()) { option.setUnit(PtlOption.UNIT.UNIT_MM); string fontName = "メイリオ"; float fontSize = 24.0f; using (PtlParamFont fontNormal = new PtlParamFont(fontName, fontSize, false, false, true)) using (PtlParamFont fontBoldItalic = new PtlParamFont(fontName, fontSize, true, true, true)) using (PtlPages pages = doc.getPages()) using (PtlPage newpage = new PtlPage()) // 新しいページ using (PtlRect rectA4 = new PtlRect(0.0f, 0.0f, 297.0f, 210.0f)) // A4横用紙 { newpage.setViewBox(rectA4); pages.append(newpage, PtlPages.INSERT_OPTION.OPTION_NONE); using (PtlSize pageSize = newpage.getSize()) using (var content = newpage.getContent()) { using (PtlRect rect = new PtlRect(10, 10, pageSize.getWidth() - 20, pageSize.getHeight() - 60)) using (PtlTextBox textBox = content.drawTextBox(rect, PtlContent.ALIGN.ALIGN_TOP_LEFT, pageSize.getWidth() - 30, pageSize.getHeight() - 70)) using (PtlParamWriteStringTextBox paramWriteString = new PtlParamWriteStringTextBox()) using (PtlParamWriteStringTextBox paramWriteStringBoldItalic = new PtlParamWriteStringTextBox()) { paramWriteString.setFont(fontNormal); paramWriteString.setUnderline(true); string text1 = ("下線はPtlParamWriteStringTextBoxで指定して書くことができます。"); textBox.writeStringNL(text1, paramWriteString); paramWriteString.setUnderline(false); textBox.writeNL(); paramWriteString.setStrikeOut(true); string text2 = ("取り消し線はPtlParamWriteStringTextBoxで指定して書くことができます。"); textBox.writeStringNL(text2, paramWriteString); paramWriteString.setStrikeOut(false); textBox.writeNL(); paramWriteStringBoldItalic.setFont(fontBoldItalic); string text3 = ("太字、斜体のフォントスタイルはPtlParamFontで設定します。"); textBox.writeStringNL(text3, paramWriteStringBoldItalic); textBox.terminate(); } using (PtlColorDeviceRGB colorBack = new PtlColorDeviceRGB(1.0f, 0.8f, 0.8f)) using (PtlColorDeviceRGB colorRed = new PtlColorDeviceRGB(1.0f, 0.0f, 0.0f)) using (PtlRect rect = new PtlRect(130, 10, pageSize.getWidth() - 10, pageSize.getHeight() - 150)) using (PtlTextBox textBox2 = content.drawTextBox(rect, PtlContent.ALIGN.ALIGN_TOP_LEFT, pageSize.getWidth() - 140, pageSize.getHeight() - 160)) using (PtlParamWriteStringTextBox paramWriteString = new PtlParamWriteStringTextBox()) using (PtlParamWriteStringTextBox paramWriteStringBoldItalic = new PtlParamWriteStringTextBox()) { textBox2.setBackColor(colorBack); // 背景色を設定 textBox2.setOutlineColor(colorRed); // テキストボックスの縁取り色を設定 textBox2.setOpacity(0.6f); // 不透明度を設定 textBox2.fitToBBox(false); // TextBoxのサイズをテキストに合わせるかどうか(true:合わせる) paramWriteString.setFont(fontNormal); string text1 = ("枠で囲った文字列(背景色あり)は PtlTextBox に色を指定して書くことができます。"); textBox2.writeStringNL(text1, paramWriteString); textBox2.terminate(); } } doc.save(output); Console.WriteLine("-- 完了 --"); } } } catch (PtlException pex) { Console.WriteLine(pex.getErrorCode() + " : " + pex.getErrorMessageJP()); pex.Dispose(); } catch (Exception ex) { Console.WriteLine(ex.Message); } } } }